Notice
Recent Posts
Recent Comments
Link
투케이2K
474. (Android) [Android Native Library] [NDK] [JNI] [cpp] 안드로이드 에서 C++ 코드 사용 방법 - CMake 모듈 생성 방법 본문
Android
474. (Android) [Android Native Library] [NDK] [JNI] [cpp] 안드로이드 에서 C++ 코드 사용 방법 - CMake 모듈 생성 방법
투케이2K 2023. 2. 11. 10:37[개발 환경 설정]
개발 툴 : AndroidStudio

[방법 설명]
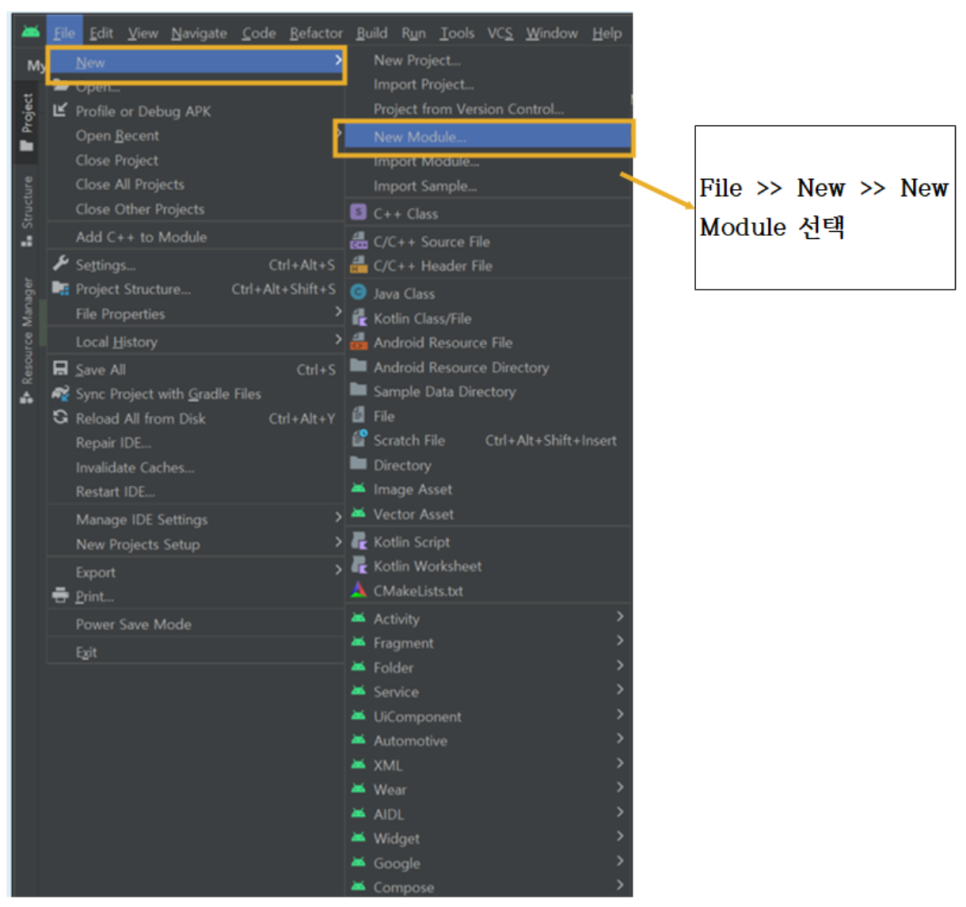
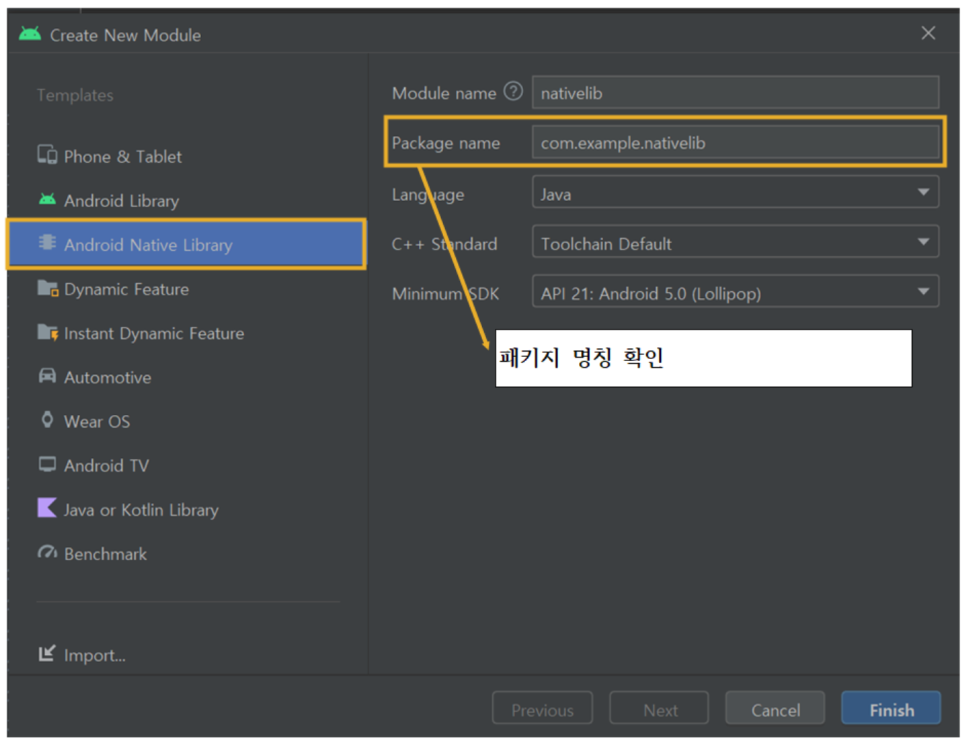
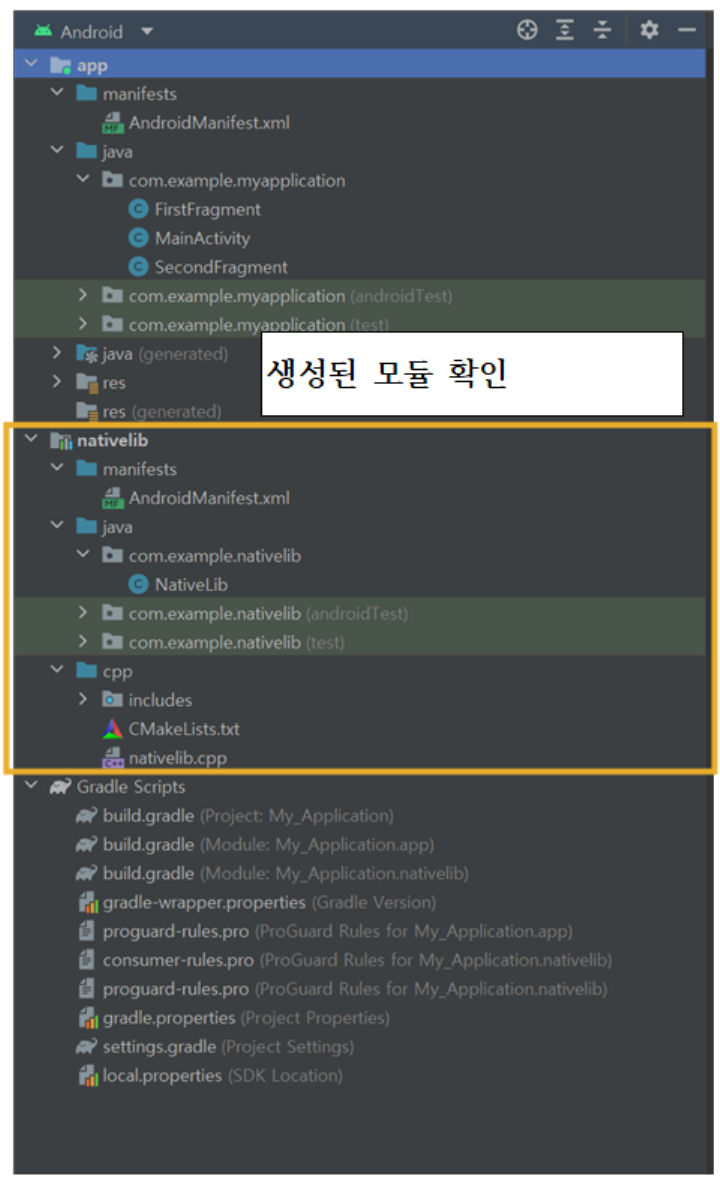
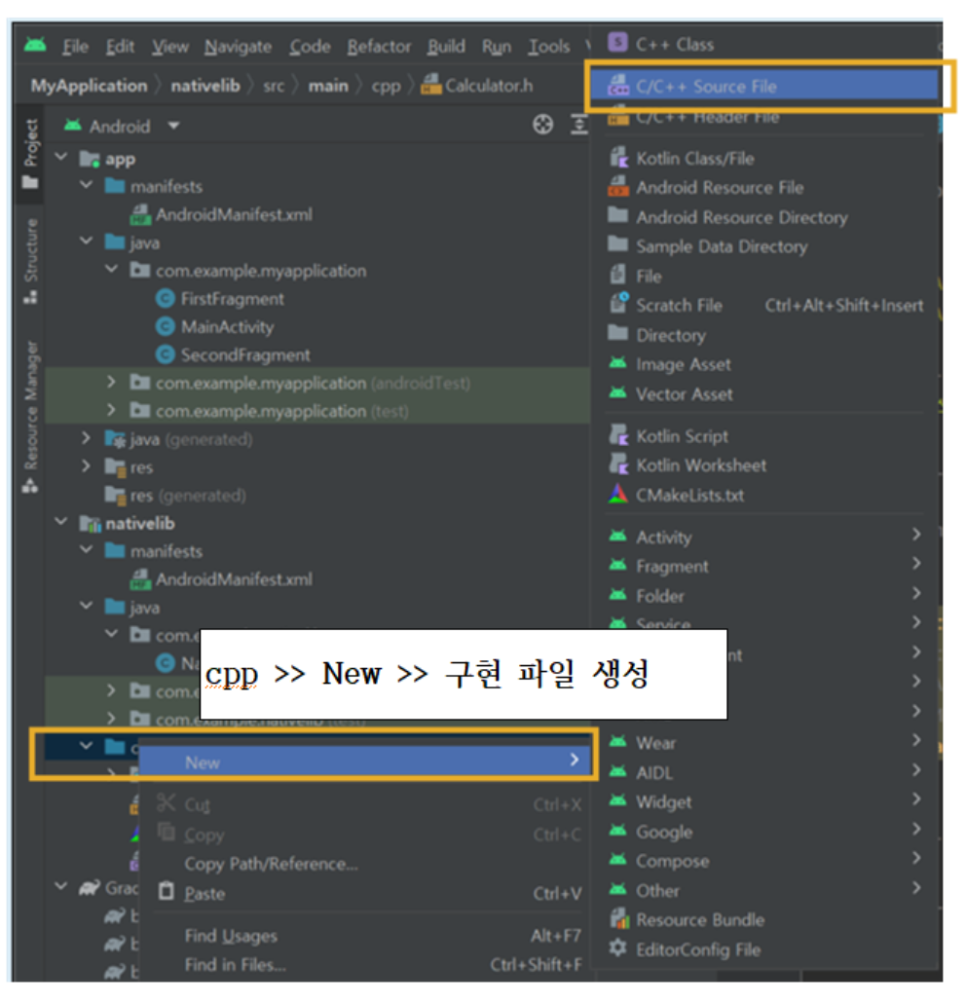
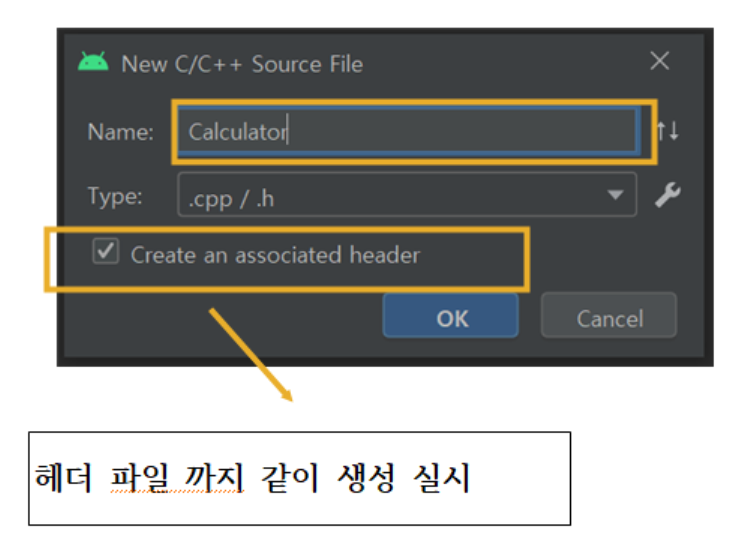
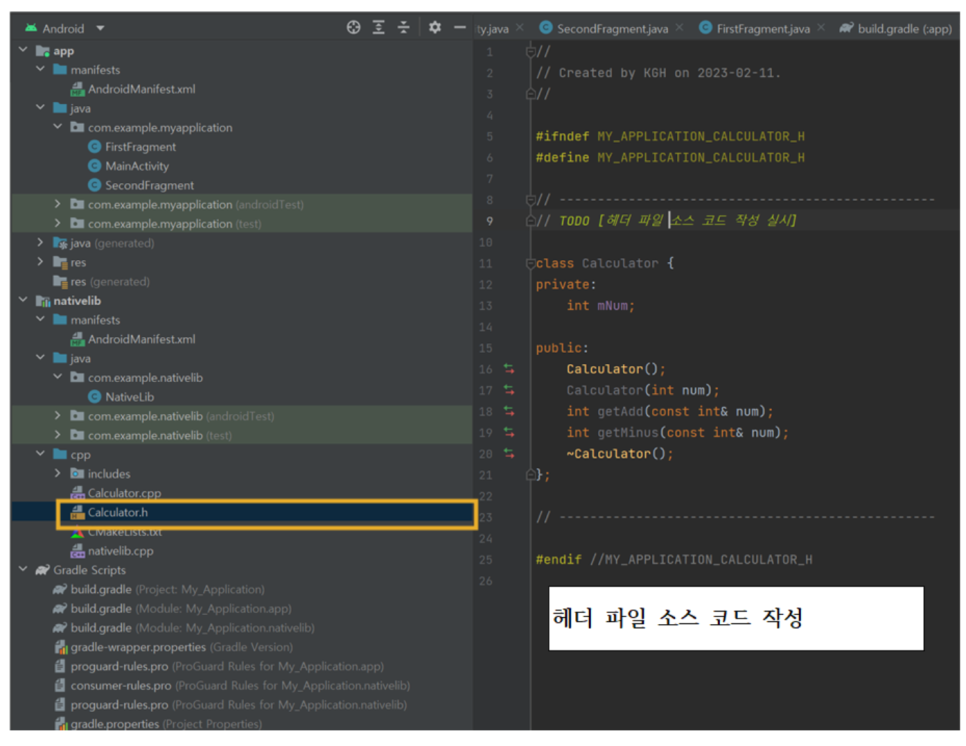
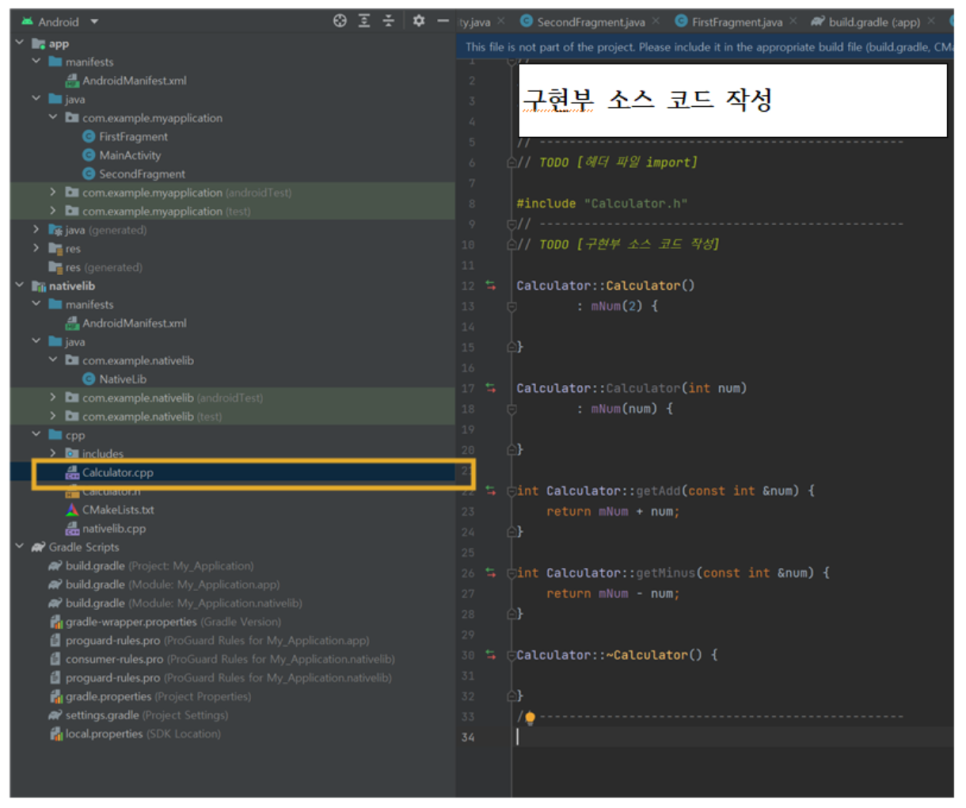
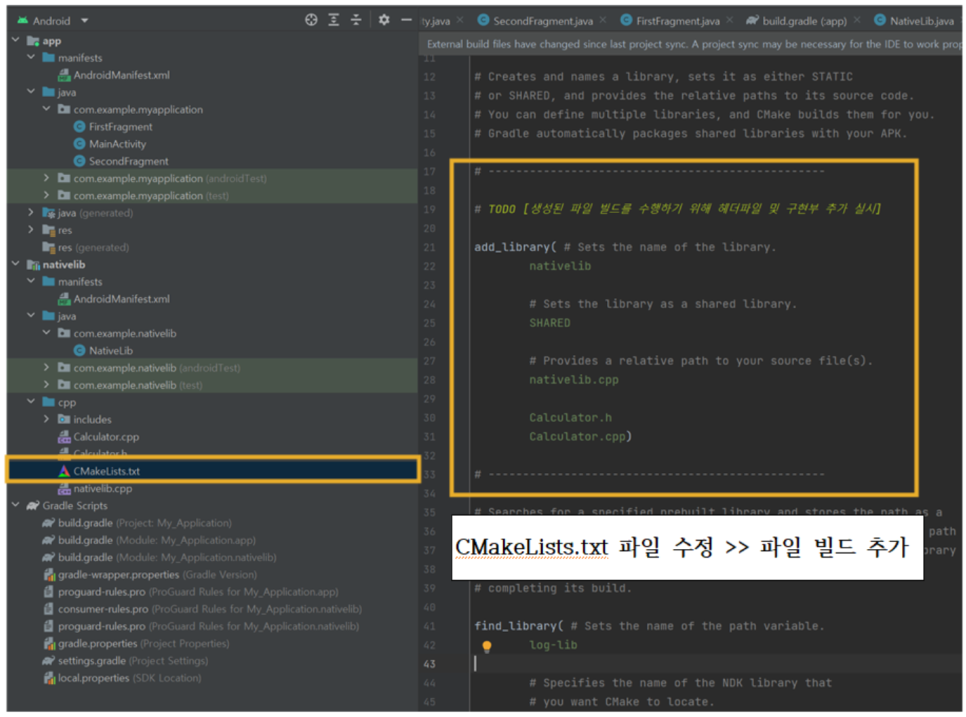
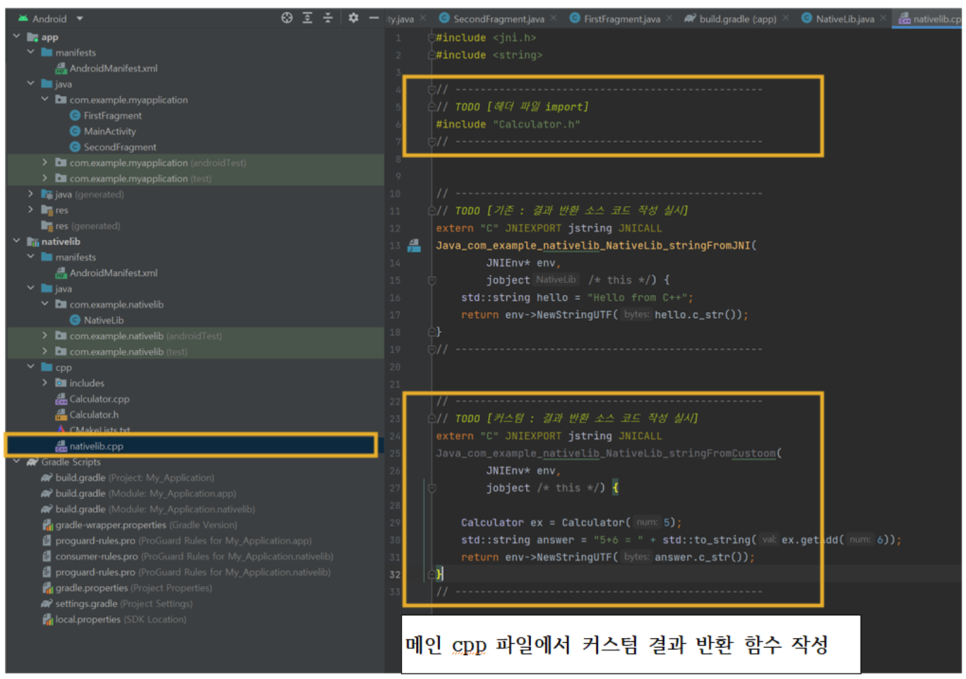
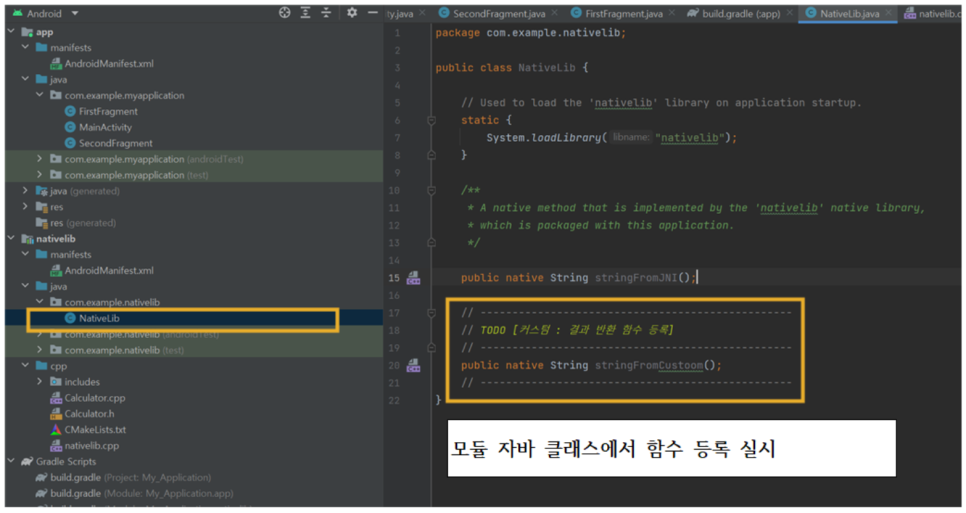
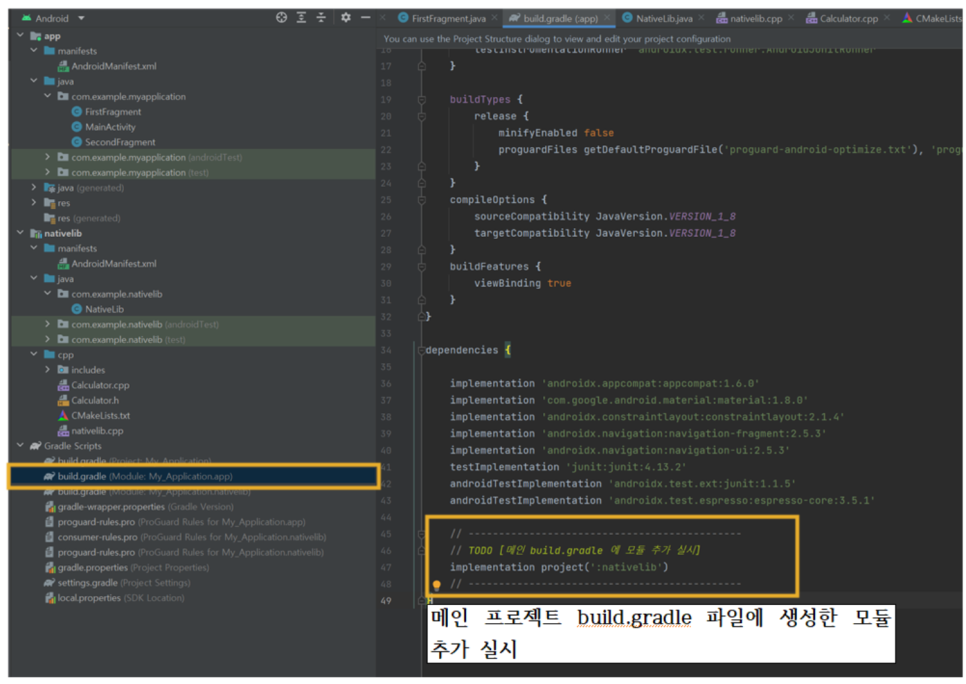
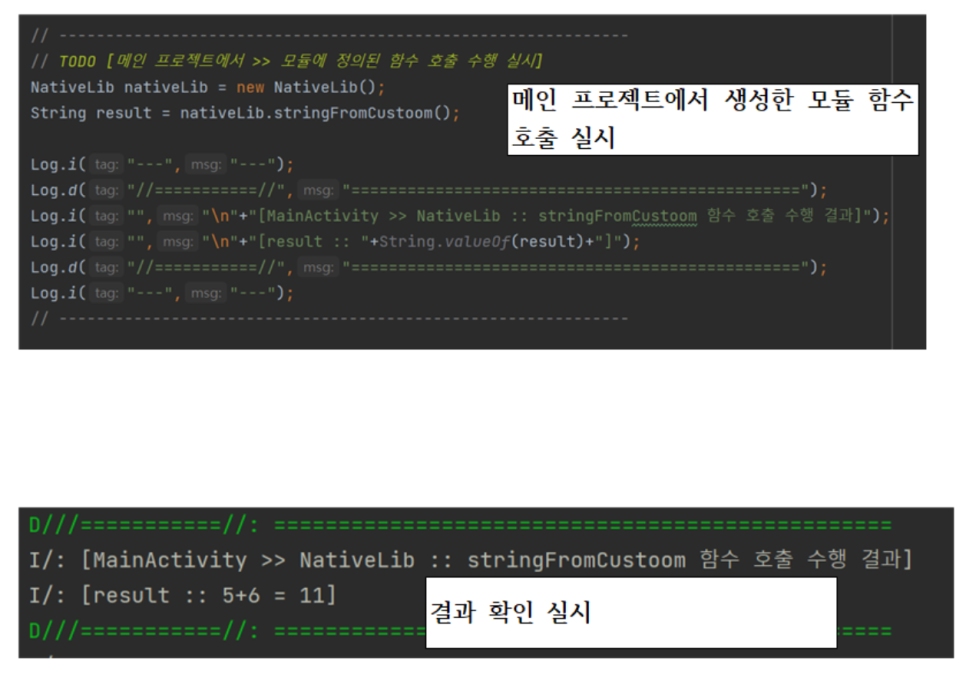
[Calculator.h : 소스 코드]
//
// Created by KGH on 2023-02-11.
//
#ifndef MY_APPLICATION_CALCULATOR_H
#define MY_APPLICATION_CALCULATOR_H
// -------------------------------------------------
// TODO [헤더 파일 소스 코드 작성 실시]
class Calculator {
private:
int mNum;
public:
Calculator();
Calculator(int num);
int getAdd(const int& num);
int getMinus(const int& num);
~Calculator();
};
// -------------------------------------------------
#endif //MY_APPLICATION_CALCULATOR_H
[Calculator.cpp : 소스 코드]
//
// Created by KGH on 2023-02-11.
//
// -------------------------------------------------
// TODO [헤더 파일 import]
#include "Calculator.h"
// -------------------------------------------------
// TODO [구현부 소스 코드 작성]
Calculator::Calculator()
: mNum(2) {
}
Calculator::Calculator(int num)
: mNum(num) {
}
int Calculator::getAdd(const int &num) {
return mNum + num;
}
int Calculator::getMinus(const int &num) {
return mNum - num;
}
Calculator::~Calculator() {
}
// -------------------------------------------------
[CMakeLists.txt : 소스 코드]
# For more information about using CMake with Android Studio, read the
# documentation: https://d.android.com/studio/projects/add-native-code.html
# Sets the minimum version of CMake required to build the native library.
cmake_minimum_required(VERSION 3.18.1)
# Declares and names the project.
project("nativelib")
# Creates and names a library, sets it as either STATIC
# or SHARED, and provides the relative paths to its source code.
# You can define multiple libraries, and CMake builds them for you.
# Gradle automatically packages shared libraries with your APK.
# -------------------------------------------------
# TODO [생성된 파일 빌드를 수행하기 위해 헤더파일 및 구현부 추가 실시]
add_library( # Sets the name of the library.
nativelib
# Sets the library as a shared library.
SHARED
# Provides a relative path to your source file(s).
nativelib.cpp
Calculator.h
Calculator.cpp)
# -------------------------------------------------
# Searches for a specified prebuilt library and stores the path as a
# variable. Because CMake includes system libraries in the search path by
# default, you only need to specify the name of the public NDK library
# you want to add. CMake verifies that the library exists before
# completing its build.
find_library( # Sets the name of the path variable.
log-lib
# Specifies the name of the NDK library that
# you want CMake to locate.
log)
# Specifies libraries CMake should link to your target library. You
# can link multiple libraries, such as libraries you define in this
# build script, prebuilt third-party libraries, or system libraries.
target_link_libraries( # Specifies the target library.
nativelib
# Links the target library to the log library
# included in the NDK.
${log-lib})
[nativelib.cpp : 소스 코드]
#include <jni.h>
#include <string>
// -------------------------------------------------
// TODO [헤더 파일 import]
#include "Calculator.h"
// -------------------------------------------------
// -------------------------------------------------
// TODO [기존 : 결과 반환 소스 코드 작성 실시]
extern "C" JNIEXPORT jstring JNICALL
Java_com_example_nativelib_NativeLib_stringFromJNI(
JNIEnv* env,
jobject /* this */) {
std::string hello = "Hello from C++";
return env->NewStringUTF(hello.c_str());
}
// -------------------------------------------------
// -------------------------------------------------
// TODO [커스텀 : 결과 반환 소스 코드 작성 실시]
// [기본 경로 [패키지 및 클래스 명칭] : Java_com_example_nativelib_NativeLib_]
// [커스텀 설정 명칭] : stringFromCustoom]
extern "C" JNIEXPORT jstring JNICALL
Java_com_example_nativelib_NativeLib_stringFromCustoom(
JNIEnv* env,
jobject /* this */) {
Calculator ex = Calculator(5);
std::string answer = "5+6 = " + std::to_string(ex.getAdd(6));
return env->NewStringUTF(answer.c_str());
}
// -------------------------------------------------
[NativeLib : 소스 코드]
package com.example.nativelib;
public class NativeLib {
// Used to load the 'nativelib' library on application startup.
static {
System.loadLibrary("nativelib");
}
/**
* A native method that is implemented by the 'nativelib' native library,
* which is packaged with this application.
*/
public native String stringFromJNI();
// -------------------------------------------------
// TODO [커스텀 : 결과 반환 함수 등록]
// -------------------------------------------------
public native String stringFromCustoom();
// -------------------------------------------------
}
[실제 모듈 함수 클래스 호출 : 소스 코드]
// -------------------------------------------------------------
// TODO [메인 프로젝트에서 >> 모듈에 정의된 함수 호출 수행 실시]
NativeLib nativeLib = new NativeLib();
String result = nativeLib.stringFromCustoom();
Log.i("---","---");
Log.d("//===========//","================================================");
Log.i("","\n"+"[MainActivity >> NativeLib :: stringFromCustoom 함수 호출 수행 결과]");
Log.i("","\n"+"[result :: "+String.valueOf(result)+"]");
Log.d("//===========//","================================================");
Log.i("---","---");
// -------------------------------------------------------------
[Module build gradle : 소스 코드]
plugins {
id 'com.android.library'
}
android {
namespace 'com.example.nativelib'
compileSdk 32
defaultConfig {
minSdk 21
targetSdk 32
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
consumerProguardFiles "consumer-rules.pro"
externalNativeBuild {
cmake {
//arguments "-DANDROID_STL=c++_static"
//cppFlags "-std=c++11 -fexceptions"
cppFlags ""
}
}
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
externalNativeBuild {
cmake {
path "src/main/cpp/CMakeLists.txt"
version "3.18.1"
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
dependencies {
implementation 'androidx.appcompat:appcompat:1.6.0'
implementation 'com.google.android.material:material:1.8.0'
testImplementation 'junit:junit:4.13.2'
androidTestImplementation 'androidx.test.ext:junit:1.1.5'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.5.1'
}
[Main build gradle : 소스 코드]
// ---------------------------------------------
// TODO [메인 build.gradle 에 모듈 추가 실시]
implementation project(':nativelib')
// ---------------------------------------------
반응형
'Android' 카테고리의 다른 글
Comments