Notice
Recent Posts
Recent Comments
Link
투케이2K
332. (AndroidStudio/android/java) 유닛 테스트 (Unit Test) 수행 방법 설명 본문
[개발 환경 설정]
개발 툴 : AndroidStudio
개발 언어 : java
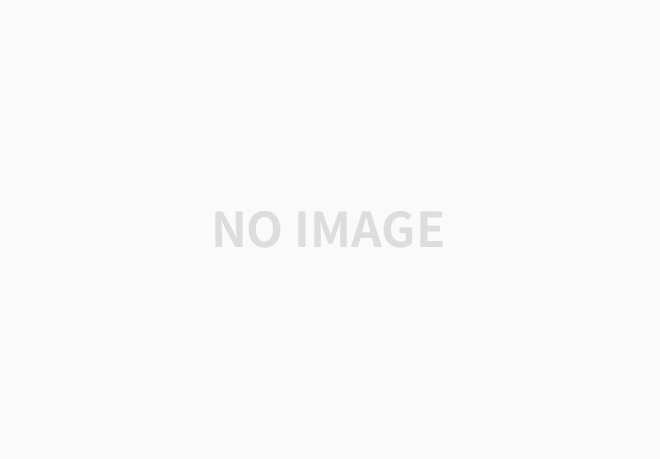
[유닛 테스트 설명]
/**
* // -----------------------------------------
* TODO [유닛 테스트 설명 및 사용 이유]
* // -----------------------------------------
* 1. 유닛 테스트는 프로그래밍에서 소스 코드의 특정 모듈이 의도된 대로 정확히 작동하는지 검증하는 절차입니다
* // -----------------------------------------
* 2. 유닛 테스트는 모든 함수와 메소드에 대한 테스트 케이스(Test case)를 작성하는 절차입니다
* // -----------------------------------------
* 3. 유닛 테스트는 필요한 부분만 테스트를 수행할 수 있으므로 전체 빌드 후 테스트를 수행하는 방법 보다 시간을 단축할 수 있습니다
* // -----------------------------------------
* 4. 유닛 단위는 메소드 및 클래스 등이 될 수 있습니다
* // -----------------------------------------
* 5. 전체 프로젝트 시간이 짧은 경우 유닛 테스트를 생략하는 경우가 많지만 추후 리팩토링을 고려해서 유닛 테스트를 진행하면서 개발하는 것이 좋습니다
* // -----------------------------------------
* */
[그림 설명]
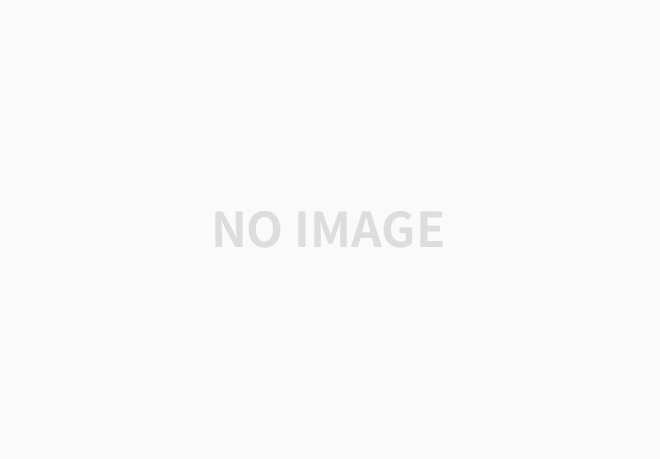
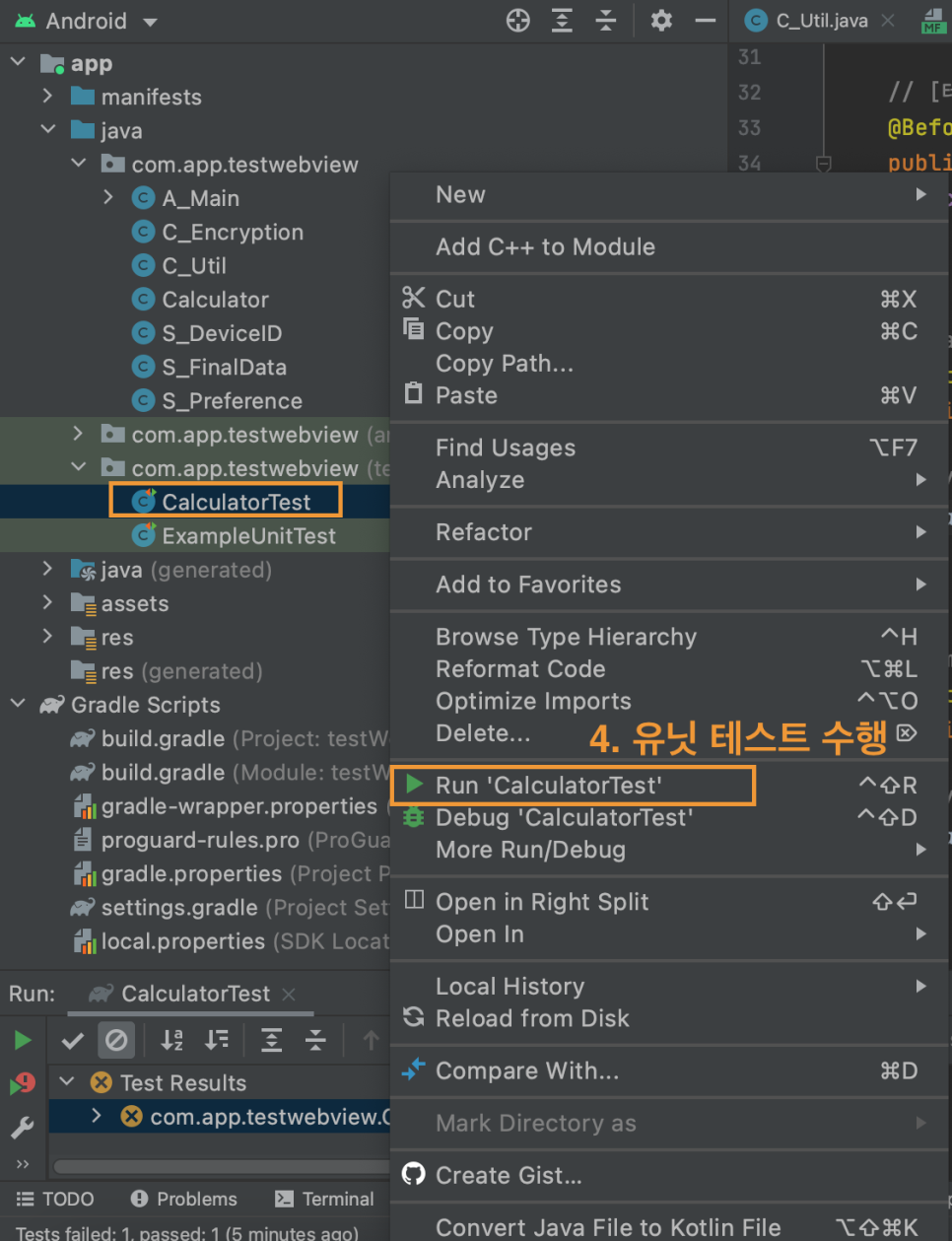
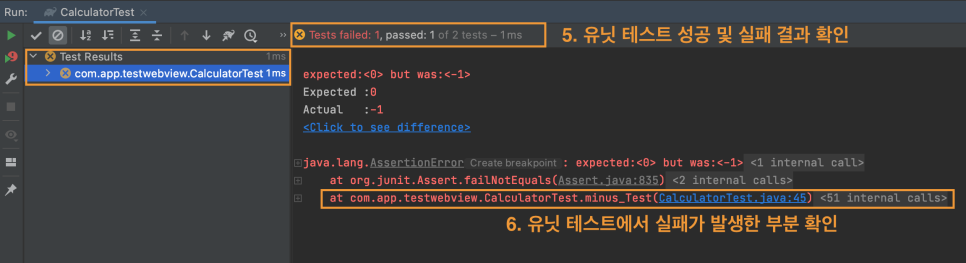
[Calcurator : 소스 코드]
package com.app.testwebview;
public class Calculator {
/**
* // -----------------------------------------
* TODO [클래스 설명]
* // -----------------------------------------
* 1. 더하기 (add), 빼기 (minus) 연산 수행 계산 클래스
* // -----------------------------------------
* 2. 유닛 테스트 작성 : CalculatorTest
* // -----------------------------------------
* */
// [더하기 수행 메소드 정의]
public int add (int one, int two){
return one + two;
}
// [빼기 수행 메소드 정의]
public int minus (int one, int two){
return one - two;
}
} // [클래스 종료]
[CalcuratorTest : 소스 코드]
package com.app.testwebview;
import static org.junit.Assert.assertEquals;
import org.junit.Before;
import org.junit.Test;
public class CalculatorTest {
/**
* // -----------------------------------------
* TODO [클래스 설명]
* // -----------------------------------------
* 1. Calculator 클래스 유닛 테스트 클래스
* // -----------------------------------------
* 2. Before : 테스트 시작 전 정의 필요 (객체 초기화)
* // -----------------------------------------
* 3. Test : 테스트로 실행되어야 하는 동작 (테스트 케이스 코드)
* // -----------------------------------------
* 4. assertEquals(예상 기대 결과, 메소드 지정 및 파라미터 삽입);
* // -----------------------------------------
* 5. 성공 (passed) / 실패 (failed) 표시
* // -----------------------------------------
* */
// [전역 변수 정의]
private Calculator calculator;
// [테스트 시작 전 정의]
@Before
public void setUp() throws Exception {
calculator = new Calculator();
}
// [add 더하기 테스트]
@Test
public void add_Test(){
// [정상 동작 >> 성공 (passed) 가 표시되는 지 확인]
assertEquals(5, calculator.add(2, 3));
}
// [minus 빼기 테스트]
@Test
public void minus_Test(){
// [에러 발생 작성 >> 에러가 표시되는지 확인]
assertEquals(0, calculator.minus(2, 3));
}
}
[build.gradle (Module) : 소스 코드]
plugins {
id 'com.android.application'
}
android {
compileSdk 30
defaultConfig {
applicationId "com.app.testwebview"
minSdk 21
targetSdk 30
versionCode 14
versionName "1.1.2"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
dependencies {
implementation 'androidx.appcompat:appcompat:1.3.1'
implementation 'com.google.android.material:material:1.4.0'
implementation 'androidx.constraintlayout:constraintlayout:2.1.1'
testImplementation 'junit:junit:4.13.2' // TODO [유닛 테스트]
androidTestImplementation 'androidx.test.ext:junit:1.1.3' // TODO [유닛 테스트]
androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0' // TODO [유닛 테스트]
}
반응형