Notice
Recent Posts
Recent Comments
Link
투케이2K
68. (spring/스프링) 스프링 부트 MSSQL SQL Server 데이터베이스 연동 방법 정의 본문
[개발 환경 설정]
개발 툴 : inteli j
개발 언어 : spring
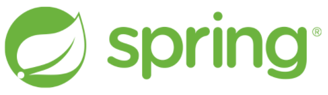
[MSSQL 접속 정보]


[프로젝트 생성 정보]
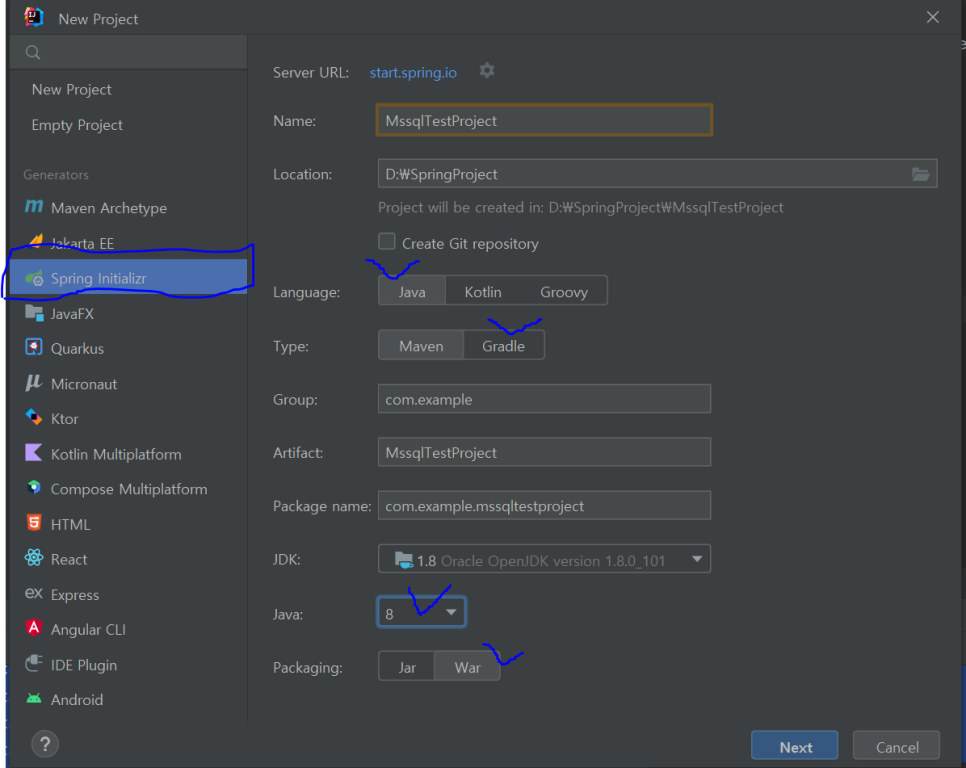

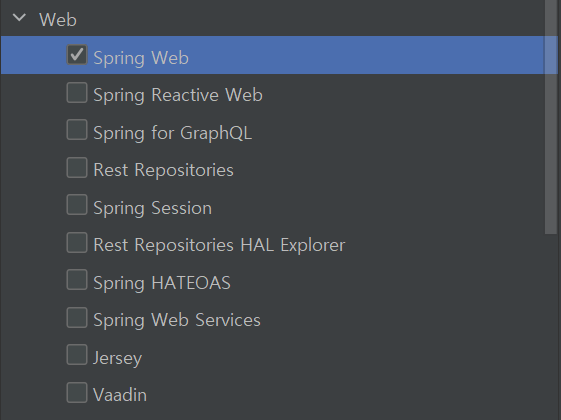
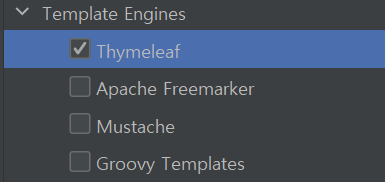
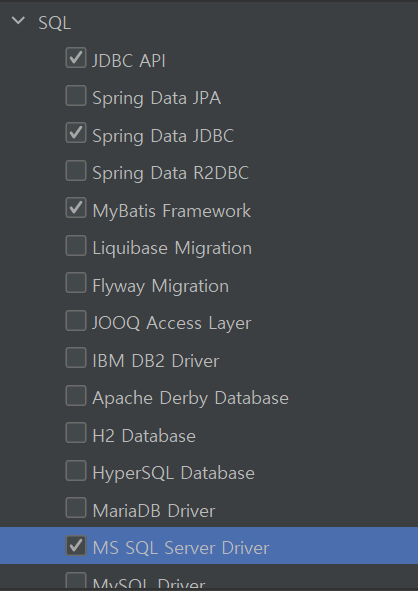
[프로젝트 구성]
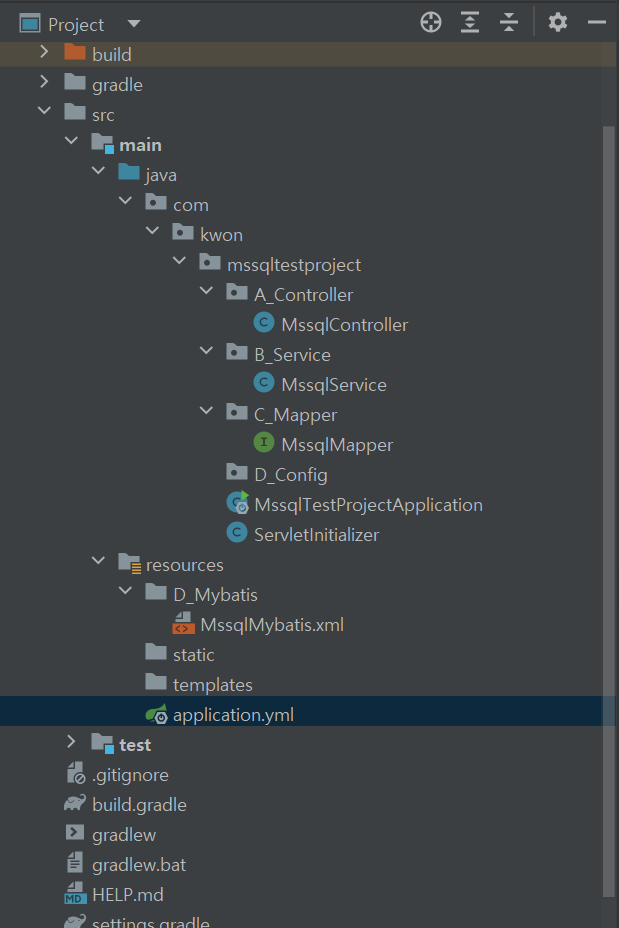
[Controller : 소스 코드]
package com.kwon.mssqltestproject.A_Controller;
import com.kwon.mssqltestproject.B_Service.MssqlService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.util.Map;
// TODO [크로스 도메인 접속 허용 설정]
@CrossOrigin("*")
// TODO [RestController / Api 처리]
@RestController
public class MssqlController {
/**
* // -----------------------------------------
* TODO [클래스 설명]
* // -----------------------------------------
* 1. 데이터베이스 접근 및 컨트롤러 클래스
* // -----------------------------------------
* 2. 사용자가 api 호출을 통해서 접근 수행
* // -----------------------------------------
* 3. service 클래스 호출 및 로직 연결 실시
* // -----------------------------------------
* */
/**
* // -----------------------------------------
* // TODO [빠른 로직 찾기 : 주석 로직 찾기]
* // -----------------------------------------
* // [SEARCH FAST] : [테스트 DB 호출]
* // -----------------------------------------
* */
// TODO [Autowire 설정]
@Autowired
private MssqlService mssqlService;
// TODO [전역 변수 선언 실시]
private static final String CLASS_NAME = "MssqlController";
// TODO [SEARCH FAST] : [테스트 DB 호출]
@GetMapping("/testDate")
public String testDate(@RequestParam Map<String, String> param){
System.out.println("\n");
System.out.println("================================================");
System.out.println("[CLASS] : "+String.valueOf(CLASS_NAME));
System.out.println("[METHOD] : "+String.valueOf("testDate"));
System.out.println("[INPUT] : "+String.valueOf(param.toString()));
System.out.println("================================================");
System.out.println("\n");
/**
* // -----------------------------------------
* [호출 방법]
* // -----------------------------------------
* 1. 호출 방식 : GET
* // -----------------------------------------
* 2. 호출 방법 : http://localhost:7000/testDate
* // -----------------------------------------
* 3. 리턴 데이터 : 2022-10-03 13:58:51.82
* // -----------------------------------------
* */
// [service 호출 수행]
String time = mssqlService.testDate();
// [Api 리턴 메시지 반환]
return time;
}
} // [클래스 종료]
[Service : 소스 코드]
package com.kwon.mssqltestproject.B_Service;
import com.kwon.mssqltestproject.C_Mapper.MssqlMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
// TODO [서비스 어노테이션]
@Service
public class MssqlService {
/**
* // -----------------------------------------
* TODO [클래스 설명]
* // -----------------------------------------
* 1. 데이터베이스 접근 및 서비스 클래스
* // -----------------------------------------
* 2. mapper 인터페이스 메소드 호출 수행 실시
* // -----------------------------------------
* */
/**
* // -----------------------------------------
* // TODO [빠른 로직 찾기 : 주석 로직 찾기]
* // -----------------------------------------
* // [SEARCH FAST] : [테스트 DB 호출]
* // -----------------------------------------
* */
// TODO [Autowire 설정]
@Autowired
private MssqlMapper mssqlMapper;
// TODO [SEARCH FAST] : [테스트 DB 호출]
public String testDate(){
// [mapper 인터페이스 호출 실시]
return mssqlMapper.testDate();
}
} // [클래스 종료]
[Mapper : 소스 코드]
package com.kwon.mssqltestproject.C_Mapper;
import org.apache.ibatis.annotations.Mapper;
// TODO [매퍼 어노테이션]
@Mapper
public interface MssqlMapper {
/**
* // -----------------------------------------
* TODO [클래스 설명]
* // -----------------------------------------
* 1. 데이터베이스 접근 및 매퍼 인터페이스
* // -----------------------------------------
* 2. 데이터베이스 쿼리문 수행 xml 리턴 값 받음
* // -----------------------------------------
* */
/**
* // -----------------------------------------
* // TODO [빠른 로직 찾기 : 주석 로직 찾기]
* // -----------------------------------------
* // [SEARCH FAST] : [테스트 DB 호출]
* // -----------------------------------------
* */
// TODO [SEARCH FAST] : [테스트 DB 호출]
String testDate();
} // [클래스 종료]
[Mybatis : 소스 코드]
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!-- mapper 인터페이스 지정 -->
<mapper namespace="com.kwon.mssqltestproject.C_Mapper.MssqlMapper">
<!--
// =========================================
// [파일 설명]
// =========================================
1. DB 접근 및 쿼리, 프로시저, 함수 호출 수행 파일
// =========================================
2. mapper 리턴 데이터를 받을 인터페이스 설정 필요
// =========================================
-->
<!--
// =========================================
// [빠른 로직 찾기 : 주석 로직 찾기]
// =========================================
// [SEARCH FAST] : [테스트 DB 호출]
// =========================================
-->
<!--
// =========================================
// [SEARCH FAST] : [테스트 DB 호출]
// =========================================
// [select id] [mapper 인터페이스 지정 메소드] : testDate
// =========================================
-->
<select id="testDate" resultType="String">
<![CDATA[
select getdate()
]]>
</select>
</mapper>
[application.yml : 소스 코드]
spring:
devtools:
livereload:
enabled: true
datasource:
driver-class-name: com.microsoft.sqlserver.jdbc.SQLServerDriver
url: jdbc:sqlserver://localhost:1433;serverName=DESKTOP-QDR03FS;encrypt=true;trustServerCertificate=true
username: KGH_DB
password: twok@2
server:
port: 7000
mybatis:
mapper-locations: classpath:D_Mybatis/*.xml
[build.gradle : 소스 코드]
plugins {
id 'org.springframework.boot' version '2.7.4'
id 'io.spring.dependency-management' version '1.0.14.RELEASE'
id 'java'
id 'war'
}
group = 'com.kwon'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '1.8'
//configurations {
// compileOnly {
// extendsFrom annotationProcessor
// }
//}
// TODO [war 파일 빌드 명칭 변경]
war {
archiveName 'mssql_test.war'
}
repositories {
mavenCentral()
}
// TODO [war file create]
configurations {
developmentOnly
runtimeClasspath {
extendsFrom developmentOnly
}
compileOnly {
extendsFrom annotationProcessor
}
}
dependencies {
// TODO [spring jdbc and settings]
implementation 'org.springframework.boot:spring-boot-starter-data-jdbc'
implementation 'org.springframework.boot:spring-boot-starter-jdbc'
implementation 'org.springframework.boot:spring-boot-starter-thymeleaf'
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.mybatis.spring.boot:mybatis-spring-boot-starter:2.2.2'
compileOnly 'org.projectlombok:lombok'
developmentOnly 'org.springframework.boot:spring-boot-devtools'
// TODO [mssql database]
runtimeOnly 'com.microsoft.sqlserver:mssql-jdbc'
// TODO [implement]
annotationProcessor 'org.springframework.boot:spring-boot-configuration-processor'
annotationProcessor 'org.projectlombok:lombok'
providedRuntime 'org.springframework.boot:spring-boot-starter-tomcat'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
tasks.named('test') {
useJUnitPlatform()
}
[결과 출력]
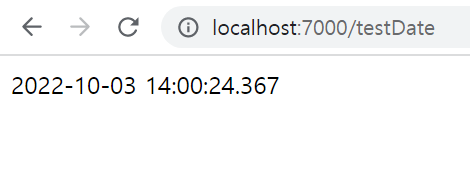
반응형
'Spring' 카테고리의 다른 글
Comments