Notice
Recent Posts
Recent Comments
Link
투케이2K
357. (ios/swift5) [유틸 파일] HTTP 쿠키 값을 자바스크립트 document.cookie 쿠키 스트링 값으로 포맷 실시 본문
IOS
357. (ios/swift5) [유틸 파일] HTTP 쿠키 값을 자바스크립트 document.cookie 쿠키 스트링 값으로 포맷 실시
투케이2K 2023. 10. 28. 00:30[개발 환경 설정]
개발 툴 : XCODE
개발 언어 : SWIFT5
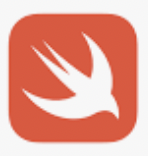
[소스 코드]
// -----------------------------------------------------------------------------------------s
// MARK: - [HTTP 쿠키 값을 document cookie 값으로 포맷 실시]
// -----------------------------------------------------------------------------------------
func getHttpCookieToCookieString(cookie : HTTPCookie?) -> String {
/*
// -----------------------------------------
[getHttpCookieToCookieString 메소드 설명]
// -----------------------------------------
1. HTTP 쿠키 값을 document cookie 값으로 포맷 실시
// -----------------------------------------
2. 호출 방법 :
// [HTTPCookie 변수 선언]
let authCookie = HTTPCookie(properties: [
.domain: "twok.com",
.path: "/",
.name: "TSEESION",
.value: "ABCD1234567890",
.sameSitePolicy: "None",
.secure: "TRUE"
])
// [메소드 호출]
C_Util().getHttpCookieToCookieString(cookie: authCookie)
// -----------------------------------------
3. 리턴 반환 :
document.cookie='version=0; TSEESION=ABCD1234567890; expires='(null)'; created='2023-10-27 15:05:33 +0000'; SessionOnly=true; domain=twok.com; HTTPOnly=false; partition=none; SameSite=none; path=/; Secure=true;'
// -----------------------------------------
*/
// [리턴 변수 선언]
var returnData = ""
// [사전 널 체크 수행]
if cookie != nil {
// ------------------------------------------
returnData = "document.cookie='"
// ------------------------------------------
if cookie?.version != nil && String(describing: cookie?.version.description ?? "").count > 0 {
returnData += "version=\(cookie?.version.description ?? ""); "
}
// ------------------------------------------
if cookie?.name != nil && String(describing: cookie?.name.description ?? "").count > 0
&& cookie?.value != nil && String(describing: cookie?.value.description ?? "").count > 0 {
returnData += "\(cookie?.name ?? "")=\(cookie?.value ?? ""); "
}
// ------------------------------------------
if cookie != nil && String(describing: cookie?.description ?? "").count > 0
&& String(describing: cookie?.description ?? "").lowercased().replacingOccurrences(of: " ", with: "").contains("expiresdate:") == true{
// [문자열 변환]
var data = String(describing: cookie?.description ?? "").replacingOccurrences(of: "\n\t", with: ";")
// [split 분리]
var array = data.split(separator: ";")
// [for 문 돌면서 체크 실시]
if array != nil && array.count > 0 {
for i in stride(from: 0, through: array.count-1, by: 1) {
if array[i].lowercased().contains("expiresdate") == true {
var item = array[i].lowercased().replacingOccurrences(of: ";", with: "") // [세미 콜론 제거]
var getValue = item.split(separator: ":") // [value 파싱]
if getValue != nil && getValue.count > 0 {
var date = ""
for j in stride(from: 0, through: getValue.count-1, by: 1) {
if j != 0 {
if j != getValue.count - 1 {
date += getValue[j] + ":"
}
else {
date += getValue[j]
}
}
}
if data.count > 0 {
returnData += "expires=\(date.trimmingCharacters(in: .whitespacesAndNewlines)); "
break
}
}
}
}
}
}
// ------------------------------------------
if cookie != nil && String(describing: cookie?.description ?? "").count > 0
&& String(describing: cookie?.description ?? "").lowercased().replacingOccurrences(of: " ", with: "").contains("created:") == true{
// [문자열 변환]
var data = String(describing: cookie?.description ?? "").replacingOccurrences(of: "\n\t", with: ";")
// [split 분리]
var array = data.split(separator: ";")
// [for 문 돌면서 체크 실시]
if array != nil && array.count > 0 {
for i in stride(from: 0, through: array.count-1, by: 1) {
if array[i].lowercased().contains("created") == true {
var item = array[i].lowercased().replacingOccurrences(of: ";", with: "") // [세미 콜론 제거]
var getValue = item.split(separator: ":") // [value 파싱]
if getValue != nil && getValue.count > 0 {
var date = ""
for j in stride(from: 0, through: getValue.count-1, by: 1) {
if j != 0 {
if j != getValue.count - 1 {
date += getValue[j] + ":"
}
else {
date += getValue[j]
}
}
}
if data.count > 0 {
returnData += "created=\(date.trimmingCharacters(in: .whitespacesAndNewlines)); "
break
}
}
}
}
}
}
// ------------------------------------------
if cookie?.isSessionOnly != nil && String(describing: cookie?.isSessionOnly.description ?? "").count > 0 {
returnData += "SessionOnly=\(cookie?.isSessionOnly.description ?? ""); "
}
// ------------------------------------------
if cookie?.domain != nil && String(describing: cookie?.domain.description ?? "").count > 0 {
returnData += "domain=\(cookie?.domain ?? ""); "
}
// ------------------------------------------
if cookie?.isHTTPOnly != nil && String(describing: cookie?.isHTTPOnly.description ?? "").count > 0 {
returnData += "HTTPOnly=\(cookie?.isHTTPOnly.description ?? ""); "
}
// ------------------------------------------
if cookie != nil && String(describing: cookie?.description ?? "").count > 0
&& String(describing: cookie?.description ?? "").lowercased().replacingOccurrences(of: " ", with: "").contains("partition:") == true{
// [문자열 변환]
var data = String(describing: cookie?.description ?? "").replacingOccurrences(of: "\n\t", with: ";")
// [split 분리]
var array = data.split(separator: ";")
// [for 문 돌면서 체크 실시]
if array != nil && array.count > 0 {
for i in stride(from: 0, through: array.count-1, by: 1) {
if array[i].lowercased().contains("partition") == true {
var item = array[i].lowercased().replacingOccurrences(of: ";", with: "") // [세미 콜론 제거]
var getValue = item.split(separator: ":") // [value 파싱]
if (getValue != nil && getValue.count > 0){
returnData += "partition=\(getValue[1].description.trimmingCharacters(in: .whitespacesAndNewlines)); "
break
}
}
}
}
}
// ------------------------------------------
if cookie != nil && String(describing: cookie?.description ?? "").count > 0
&& String(describing: cookie?.description ?? "").lowercased().replacingOccurrences(of: " ", with: "").contains("samesite:") == true{
// [문자열 변환]
var data = String(describing: cookie?.description ?? "").replacingOccurrences(of: "\n\t", with: ";")
// [split 분리]
var array = data.split(separator: ";")
// [for 문 돌면서 체크 실시]
if array != nil && array.count > 0 {
for i in stride(from: 0, through: array.count-1, by: 1) {
if array[i].lowercased().contains("samesite") == true {
var item = array[i].lowercased().replacingOccurrences(of: ";", with: "") // [세미 콜론 제거]
var getValue = item.split(separator: ":") // [value 파싱]
if getValue != nil && getValue.count > 0 {
returnData += "SameSite=\(getValue[1].trimmingCharacters(in: .whitespacesAndNewlines)); "
break
}
}
}
}
}
// ------------------------------------------
if cookie?.path != nil && String(describing: cookie?.path.description ?? "").count > 0 {
returnData += "path=\(cookie?.path ?? ""); "
}
// ------------------------------------------
if cookie?.isSecure != nil && String(describing: cookie?.isSecure.description ?? "").count > 0 {
returnData += "Secure=\(cookie?.isSecure.description ?? "");"
}
// ------------------------------------------
returnData += "'"
// ------------------------------------------
}
// [로그 출력 실시]
//*
S_Log._D_(description: "HTTP 쿠키 값을 document cookie 값으로 포맷 실시", data: [
"INPUT :: \(String(describing: cookie?.description ?? ""))",
"RETURN :: \(returnData)"
])
// */
// [리턴 데이터 반환 실시]
return "\(returnData)"
}
[결과 출력]
================================================================
LOG :: CLASS PLACE :: C_Util.swift :: getHttpCookieToCookieString(cookie:) :: 3262
-------------------------------------------------
LOG :: NOW TIME :: 2023-10-28 00:05:33
-------------------------------------------------
LOG :: DESCRIPTION :: HTTP 쿠키 값을 document cookie 값으로 포맷 실시
-------------------------------------------------
LOG :: INPUT :: <NSHTTPCookie
version:0
name:TSEESION
value:ABCD1234567890
expiresDate:'(null)'
created:'2023-10-27 15:05:33 +0000'
sessionOnly:TRUE
domain:twok.com
partition:none
sameSite:none
path:/
isSecure:TRUE
path:"/" isSecure:TRUE>
-------------------------------------------------
LOG :: RETURN :: document.cookie='version=0; TSEESION=ABCD1234567890; expires='(null)'; created='2023-10-27 15:05:33 +0000'; SessionOnly=true; domain=twok.com; HTTPOnly=false; partition=none; SameSite=none; path=/; Secure=true;'
================================================================
반응형
'IOS' 카테고리의 다른 글
Comments