반응형
Notice
Recent Posts
Recent Comments
Link
투케이2K
95. (Go Lang) [Mac Os] Go 문법 : net/http 모듈 (BackEnd) - http 모듈 사용해 Post 방식 Body Json 호출 실시 본문
Go Lang (Go 언어)
95. (Go Lang) [Mac Os] Go 문법 : net/http 모듈 (BackEnd) - http 모듈 사용해 Post 방식 Body Json 호출 실시
투케이2K 2024. 2. 29. 21:09반응형
[개발 환경 설정]
개발 언어 : Go
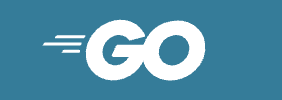
[소스 코드]
package main
import (
"encoding/json"
"fmt"
"log"
"net/http"
)
// -------------------------------------------------------------------------------
// [서버 URL Path 경로에 따른 분기 처리]
func urlPath(write http.ResponseWriter, request *http.Request) {
fmt.Println("")
fmt.Println("----------------------------------------------")
fmt.Println("[urlPath] : [Start]")
fmt.Println("----------------------------------------------")
fmt.Println("INPUT [Method] : " + request.Method)
fmt.Println("----------------------------------------------")
fmt.Println("INPUT [Path] : " + request.URL.Path)
fmt.Println("----------------------------------------------")
fmt.Println("")
// [사전 조건 체크]
if request.URL.Path == "/" {
http.Error(write, "404 not found (path)", http.StatusNotFound)
return
}
// [http 요청 타입 및 path 별 분기 처리]
switch request.Method {
case "POST":
if request.URL.Path == "/post" {
// [Body Json 데이터를 파싱 할 구조체 정의]
var user User
// [Request 요청 들어온 Body Json 데이터 확인]
err := json.NewDecoder(request.Body).Decode(&user)
if err != nil {
http.Error(write, "404 not found (json)", http.StatusNotFound)
return
}
// [구조체 정보 확인]
fmt.Println("")
fmt.Println("----------------------------------------------")
fmt.Println("[urlPath] : [Request] : [Json]")
fmt.Println("----------------------------------------------")
fmt.Println("name : " + user.Name)
fmt.Println("----------------------------------------------")
fmt.Println("")
// [리턴 JSON 정의]
response := Response{true}
// [Json 인코딩 수행 : Marshal]
jsonBytes, err := json.Marshal(response)
if err != nil {
panic(err)
}
// [Json 바이트를 문자열로 변경]
jsonString := string(jsonBytes)
// [리턴 반환]
fmt.Fprintf(write, "%s\n", jsonString)
} else {
// [에러 반환]
http.Error(write, "404 not found (get)", http.StatusNotFound)
}
default:
fmt.Println("")
fmt.Println("----------------------------------------------")
fmt.Println("[urlPath] : [Default]")
fmt.Println("----------------------------------------------")
fmt.Println("Error : Methods Are Supported")
fmt.Println("----------------------------------------------")
fmt.Println("")
// [에러 반환]
http.Error(write, "404 not found (default)", http.StatusNotFound)
}
}
// -------------------------------------------------------------------------------
// [기본 서버 구동 함수]
func main() {
// ---------------------------------------------------
// [기본 설명]
// ---------------------------------------------------
// "net/http" 패키지를 사용해 Http 통신 백엔드 서버를 구성할 수 있습니다
// ---------------------------------------------------
// ListenAnServer 함수는 TCP 네트워크에 주소에서 수신 대기(Listen)을 한 다음 통신 요청들을 받아 Server(서버) 함수를 통해 handler 함수를 실행시킵니다
// ---------------------------------------------------
// [http 핸들러 메소드 정의]
http.HandleFunc("/", urlPath)
// [http 서버 구동 수행]
if err := http.ListenAndServe(":8080", nil); err != nil {
log.Fatal(err)
}
}
// -------------------------------------------------------------------------------
// [Request BODY JSON 인코딩 구조체 생성]
type User struct {
Name string `json:"name"`
}
// -------------------------------------------------------------------------------
// [Response JSON 인코딩 구조체 생성]
type Response struct {
Success bool `json:"success"`
}
// -------------------------------------------------------------------------------
[결과 출력]
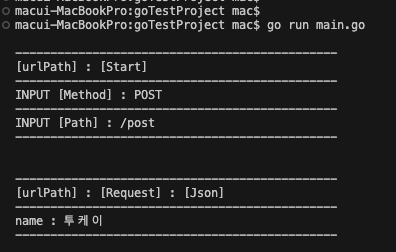
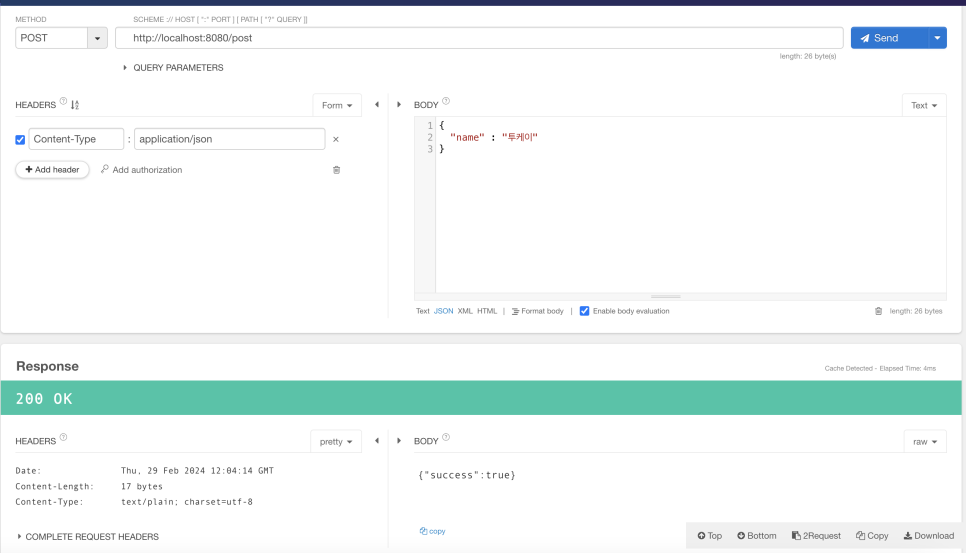
반응형
'Go Lang (Go 언어)' 카테고리의 다른 글
Comments