Notice
Recent Posts
Recent Comments
Link
투케이2K
878. (Android/Java) [유틸 파일] getSocketFactory : CA , Crt , Key 사용해 SSL 인증 시 필요한 SSLSocketFactory 반환 본문
Android
878. (Android/Java) [유틸 파일] getSocketFactory : CA , Crt , Key 사용해 SSL 인증 시 필요한 SSLSocketFactory 반환
투케이2K 2024. 9. 18. 09:37[개발 환경 설정]
개발 툴 : AndroidStudio
개발 언어 : Java / Kotlin
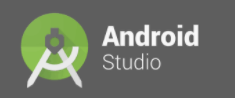
[소스 코드]
// ------------------------------------------------------------------------------------------
// TODO [SSL 인증 시 필요한 getSocketFactory 유틸 파일]
// ------------------------------------------------------------------------------------------
public static SSLSocketFactory getSocketFactory(InputStream CA_FILE, InputStream CRT_FILE, InputStream PRIVATE_KET_FILE) {
// [리턴 변수 선언]
SSLSocketFactory returnData = null;
String M_LOG = "";
// [로직 처리 수행]
try {
if (CA_FILE != null && CRT_FILE != null && PRIVATE_KET_FILE != null){
// ---------------------------------------------------
// TODO [Certificate Type] : 인증서 타입 지정
// ---------------------------------------------------
CertificateFactory cf = CertificateFactory.getInstance("X.509");
// ---------------------------------------------------
// TODO [Load CA certificate] : CA 인증서 지정
// ---------------------------------------------------
X509Certificate caCert = (X509Certificate) cf.generateCertificate(CA_FILE);
// ---------------------------------------------------
// TODO [Load client certificate] : 클라이언트 CRT 인증서 지정 (클레임 인증서)
// ---------------------------------------------------
X509Certificate clientCert = (X509Certificate) cf.generateCertificate(CRT_FILE);
// ---------------------------------------------------
// TODO [Load client private key] : 클라이언트 private key 키 파일 지정
// ---------------------------------------------------
PrivateKey privateKey = loadPrivateKey(PRIVATE_KET_FILE);
// ---------------------------------------------------
// TODO [Create a KeyStore for the client certificate and private key]
// ---------------------------------------------------
// TODO [클라이언트 인증서와 키파일을 키스토어에 저장 수행] : [Aws 의 경우 KeyStore 에 저장 된 형식이 BKS 필요]
// ---------------------------------------------------
KeyStore keyStore = KeyStore.getInstance(KeyStore.getDefaultType());
keyStore.load(null, null);
keyStore.setCertificateEntry("ca-cert", caCert);
keyStore.setKeyEntry("client-key", privateKey, "password".toCharArray(), new Certificate[]{clientCert});
// ---------------------------------------------------
// TODO [Create a TrustManager that trusts the CA in our KeyStore]
// ---------------------------------------------------
// TODO [KeyStore 에서 CA 를 신뢰하는 TrustManager 를 만듭니다]
// ---------------------------------------------------
TrustManagerFactory tmf = TrustManagerFactory.getInstance(TrustManagerFactory.getDefaultAlgorithm());
tmf.init(keyStore);
// ---------------------------------------------------
// TODO [Create a KeyManager that uses our client key and certificate]
// ---------------------------------------------------
// TODO [클라이언트 키와 인증서를 사용하는 KeyManager 를 만듭니다]
// ---------------------------------------------------
KeyManagerFactory kmf = KeyManagerFactory.getInstance(KeyManagerFactory.getDefaultAlgorithm());
kmf.init(keyStore, "password".toCharArray());
// ---------------------------------------------------
// TODO [Create an SSLContext that uses the KeyManager and TrustManager]
// ---------------------------------------------------
// TODO [KeyManager 및 TrustManager 를 사용하는 SSLContext 를 만듭니다]
// ---------------------------------------------------
SSLContext context = SSLContext.getInstance("TLSv1.2"); // [TLS 버전 지정]
context.init(kmf.getKeyManagers(), tmf.getTrustManagers(), null);
// ---------------------------------------------------
// TODO [getSocketFactory 리턴 결과 반환]
// ---------------------------------------------------
returnData = context.getSocketFactory();
M_LOG = "[Success] :: getSocketFactory";
}
else {
M_LOG = "[Error] :: getSocketFactory :: Input Data Is Null";
}
}
catch (Exception e){
e.printStackTrace();
S_Log._printStackTrace_(null, "getSocketFactory :: Exception", null, e);
M_LOG = "[Exception] :: getSocketFactory :: " + String.valueOf(e.getMessage());
}
// [로그 출력 수행]
S_Log._W_("getSocketFactory :: SSL 소켓 설정 결과 확인", new String[]{String.valueOf(M_LOG)});
// [리턴 반환 수행]
return returnData;
}
// -----------------------------------------------------------------------------------------
// TODO [SEARCH FAST] : [RETURN] loadPrivateKey : 특정 문자 제거 및 개인키 반환 수행
// -----------------------------------------------------------------------------------------
public static PrivateKey loadPrivateKey(InputStream inputStream) {
/**
* // -----------------------------------------
* [stringToInputstream 메소드 설명]
* // -----------------------------------------
* 1. 특정 문자 제거 및 개인키 반환 수행
* // -----------------------------------------
* 2. 호출 방식 :
*
* C_App.loadPrivateKey(keyFile);
*
* // -----------------------------------------
* 3. 리턴 데이터 :
*
* PrivateKey
* // -----------------------------------------
* */
// [리턴 값 선언]
PrivateKey returnData = null;
String M_LOG = "";
// [로직 처리 실시]
try {
// [인풋 데이터 체크]
if (inputStream != null){
byte[] keyBytes = new byte[inputStream.available()];
inputStream.read(keyBytes);
inputStream.close();
String privateKeyPEM = new String(keyBytes);
privateKeyPEM = privateKeyPEM.replaceAll("-----BEGIN RSA PRIVATE KEY-----", "");
privateKeyPEM = privateKeyPEM.replaceAll("-----END RSA PRIVATE KEY-----", "");
privateKeyPEM = privateKeyPEM.replaceAll("-----BEGIN PRIVATE KEY-----", "");
privateKeyPEM = privateKeyPEM.replaceAll("-----END PRIVATE KEY-----", "");
keyBytes = Base64.decode(privateKeyPEM, Base64.DEFAULT);
PKCS8EncodedKeySpec spec = new PKCS8EncodedKeySpec(keyBytes);
KeyFactory kf = KeyFactory.getInstance("RSA");
returnData = kf.generatePrivate(spec);
M_LOG = "[Success] :: Private Key File Return";
}
else {
M_LOG = "[Error] :: Input Data Is Null";
}
} catch (Exception e) {
M_LOG = "[Exception] :: " + String.valueOf(e.getMessage());
S_Log._printStackTrace_(null, S_FinalData.LOG_BUG_STATE, null, e);
}
// [로그 출력 실시]
///*
// ===============================================================
S_Log._D_("특정 문자 제거 및 개인키 반환 수행", new String[]{
"M_LOG :: " + String.valueOf(M_LOG),
"RETURN :: " + String.valueOf(returnData)
});
// ===============================================================
// */
// [리턴 반환 실시]
return returnData;
}
반응형
'Android' 카테고리의 다른 글
Comments