Notice
Recent Posts
Recent Comments
Link
투케이2K
951. (Android/Java) [간단 소스] 안드로이드 휴대폰 번호 입력 팝업창 UI 유틸 파일 제작 - 커스텀 Alert 팝업창 본문
Android
951. (Android/Java) [간단 소스] 안드로이드 휴대폰 번호 입력 팝업창 UI 유틸 파일 제작 - 커스텀 Alert 팝업창
투케이2K 2025. 2. 21. 19:56[개발 환경 설정]
개발 툴 : AndroidStudio
개발 언어 : Java / Kotlin
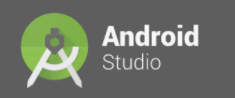
[소스 코드]
// --------------------------------------------------------------------------------------
[개발 및 테스트 환경]
// --------------------------------------------------------------------------------------
- 언어 : Java / Kotlin
- 개발 툴 : AndroidStudio
- 기술 구분 : Alert / 커스텀 / 휴대폰 번호 입력 팝업창
// --------------------------------------------------------------------------------------
// --------------------------------------------------------------------------------------
[xml 소스 코드]
// --------------------------------------------------------------------------------------
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/activity_main__door_guard__main_search_setting_state"
android:layout_width="match_parent"
android:layout_height="245dp"
android:orientation="vertical"
android:background="#ffffff"
android:focusable="true"
android:focusableInTouchMode="true">
<TextView
android:layout_width="match_parent"
android:layout_height="50dp"
android:text="회원 가입 SMS 문자 전송"
android:textStyle="bold"
android:textColor="#ffffff"
android:textSize="15sp"
android:gravity="center"
android:singleLine="true"
android:ellipsize="end"
android:background="#19295a"
android:layout_marginBottom="5dp"/>
<TextView
android:layout_width="match_parent"
android:layout_height="50dp"
android:gravity="center"
android:lineSpacingExtra="5dp"
android:text="회원 가입 SMS 문자 전송을 하기 위한\n휴대폰 번호를 입력해주세요."
android:textColor="#000000"
android:textSize="13sp"
android:textStyle="bold" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_marginTop="5dp"
android:layout_marginBottom="5dp"
android:layout_marginLeft="3dp"
android:layout_marginRight="3dp"
android:orientation="vertical"
android:background="#000">
</LinearLayout>
<TextView
android:layout_width="match_parent"
android:layout_height="50dp"
android:text="※ 휴대폰 번호 입력 시 특수 문자 없이 입력해주세요.\n휴대폰 번호 입력 예시 : 01012345678"
android:lineSpacingExtra="5dp"
android:textStyle="bold"
android:textColor="#ff0033"
android:textSize="13sp"
android:gravity="center"
android:layout_margin="5dp"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_marginTop="5dp"
android:layout_marginBottom="5dp"
android:layout_marginLeft="3dp"
android:layout_marginRight="3dp"
android:orientation="vertical"
android:background="#000">
</LinearLayout>
<EditText
android:id="@+id/phoneEditText"
android:layout_width="match_parent"
android:layout_height="50dp"
android:text=""
android:hint=""
android:textStyle="bold"
android:textColor="#19295a"
android:textSize="13sp"
android:gravity="center"
android:singleLine="true"
android:ellipsize="end"
android:padding="0dp"
android:layout_margin="2dp"
android:background="#ccc"/>
</LinearLayout>
// -----------------------------------------------------------------------------------------
// -----------------------------------------------------------------------------------------
[Java : 소스 코드]
// -----------------------------------------------------------------------------------------
// -----------------------------------------------------------------------------------------
// TODO [SEARCH FAST] : [Observable] : [휴대폰 번호 입력 커스텀 Alert 팝업창]
// -----------------------------------------------------------------------------------------
// TODO [호출 방법 소스 코드]
// -----------------------------------------------------------------------------------------
/*
try {
// [팝업창 활성 수행]
C_Ui_View.observablePhoneInput(A_Intro.this, "010-1234-5678", "전송", "닫기")
.subscribeOn(AndroidSchedulers.mainThread()) // [Observable (생성자) 로직을 IO 스레드에서 실행 : 백그라운드]
.observeOn(Schedulers.io()) // [Observer (관찰자) 로직을 메인 스레드에서 실행]
.subscribe(new Observer<String>() { // [Observable.create 타입 지정]
@Override
public void onSubscribe(@NonNull Disposable d) {
}
@Override
public void onNext(@NonNull String value) {
}
@Override
public void onError(@NonNull Throwable e) {
}
@Override
public void onComplete() {
}
});
}
catch (Exception e){
e.printStackTrace();
}
*/
// -----------------------------------------------------------------------------------------
public static Observable<String> observablePhoneInput(Context mContext, String phoneNumber, String ok, String no){
// [로직 처리 실시]
return Observable.create(subscriber -> {
try {
// [UI 생성 실시]
LayoutInflater inflater = (LayoutInflater) mContext.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View dialogView = inflater.inflate(R.layout.alert_phone_input, null); // [xml 지정]
final EditText phoneEditText = (EditText) dialogView.findViewById(R.id.phoneEditText);
String className = "";
try { className = Thread.currentThread().getStackTrace()[3].toString(); } catch (Exception ec){}
// [로그 쌓기]
try {
S_Log._fileSave_(null, true, "C_Ui_View : observablePhoneInput : 휴대폰 번호 입력 팝업창 표시", new String[]{
"CLASS : " + String.valueOf(className),
"PHONE_NUMBER : " + String.valueOf(phoneNumber),
});
}
catch (Exception ez){}
// [팝업창 생성 실시]
new Handler(Looper.getMainLooper()).postDelayed(new Runnable() {
@Override
public void run() {
AlertDialog alertDialog;
// [AlertDialog 팝업창 생성]
AlertDialog.Builder builder = new AlertDialog.Builder(mContext);
//builder.setTitle(title); //[팝업창 타이틀 지정]
builder.setIcon(R.drawable.icon); //[팝업창 아이콘 지정]
//builder.setMessage(ment); //[팝업창 내용 지정]
builder.setCancelable(false); //[외부 레이아웃 클릭시도 팝업창이 사라지지않게 설정]
builder.setView(dialogView); // TODO [뷰 지정]
builder.setPositiveButton(ok, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// -----------------------------------------
// TODO [확인 버튼 클릭 이벤트 처리]
// -----------------------------------------
}
});
builder.setNegativeButton(no, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// -----------------------------------------
try { S_Log._fileSave_(null, true, "C_Ui_View : observablePhoneInput : ["+String.valueOf(no)+"] 버튼 클릭 이벤트 발생 : " + C_Util.getSourceCodeLine(), null); } catch (Exception ez){}
// -----------------------------------------
// -----------------------------------------
// TODO [키보드 내림]
// -----------------------------------------
//*
try {
InputMethodManager imm = (InputMethodManager)mContext.getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(phoneEditText.getWindowToken(), 0);
}
catch (Exception e){}
// */
// -----------------------------------------
// -----------------------------------------
// TODO [리턴 데이터 반환]
// TODO [리턴 데이터 반환]
// -----------------------------------------
try {
subscriber.onNext("");
subscriber.onComplete();
}
catch (Exception ex){
ex.printStackTrace();
}
// -----------------------------------------
}
});
alertDialog = builder.create();
alertDialog.show();
// -------------------------------------------
// [확인 버튼 재상속 조건이 맞는 경우만 dismiss]
// -------------------------------------------
alertDialog.getButton(AlertDialog.BUTTON_POSITIVE).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// -----------------------------------------
// TODO [뷰에 표시된 정보 얻어온다] : 특수문자 Replace 처리
// -----------------------------------------
String inputPhone = phoneEditText.getText().toString();
inputPhone = inputPhone.replaceAll(":", "");
inputPhone = inputPhone.replaceAll("-", "");
inputPhone = inputPhone.replaceAll(" ", "");
inputPhone = C_Util.getRexpNumberString(inputPhone); // TODO [정규식 숫자만 지정]
// -----------------------------------------
try { S_Log._fileSave_(null, true, "C_Ui_View : observablePhoneInput : ["+String.valueOf(ok)+"] 버튼 클릭 이벤트 발생 : " + C_Util.getSourceCodeLine(), new String[]{"입력 한 휴대폰 번호 : " + String.valueOf(phoneEditText.getText().toString()), "휴대폰 번호 정규식 수행 : " + String.valueOf(inputPhone)}); } catch (Exception ez){}
// -----------------------------------------
// -----------------------------------------
// TODO [방어 로직] : 입력 정보 Null 체크
// -----------------------------------------
if (C_Util.stringNotNull(inputPhone) == false){
C_Ui_View.toastLongError(mContext, "※ 휴대폰 입력 정보가 올바르지 않습니다. (Data Is Null)\n\n"+"정규식 수행 폰 번호 : " + inputPhone);
return;
}
// -----------------------------------------
// TODO [방어 로직] : 입력 된 EditText 글자 수 확인 : 자릿수 초과
// -----------------------------------------
if (inputPhone.length() >= 15){
C_Ui_View.toastLongError(mContext, "※ 휴대폰 입력 정보가 올바르지 않습니다. (Data Length Over)\n\n" +"정규식 수행 폰 번호 : " + inputPhone);
return;
}
// -----------------------------------------
// TODO [방어 로직] : 입력 된 EditText 글자 수 확인 : 자릿수 부족
// -----------------------------------------
if (inputPhone.length() < 11){
C_Ui_View.toastLongError(mContext, "※ 휴대폰 입력 정보가 올바르지 않습니다. (Data Length Minor)\n\n" +"정규식 수행 폰 번호 : " + inputPhone);
return;
}
// -----------------------------------------
// TODO [리턴 데이터 반환]
// -----------------------------------------
try {
subscriber.onNext(inputPhone);
subscriber.onComplete();
}
catch (Exception ex){
ex.printStackTrace();
}
// -----------------------------------------
// -----------------------------------------
// TODO [키보드 내림]
// -----------------------------------------
//*
try {
InputMethodManager imm = (InputMethodManager)mContext.getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(phoneEditText.getWindowToken(), 0);
}
catch (Exception e){}
// */
// -----------------------------------------
// -----------------------------------------
// TODO [Alert 팝업창 닫기]
// -----------------------------------------
if (alertDialog != null){
alertDialog.dismiss();
}
// -----------------------------------------
}
});
}
}, 0);
} catch (final Exception e){
e.printStackTrace();
// ------------------------------------------------------
// TODO [리턴 데이터 반환]
// ------------------------------------------------------
try {
subscriber.onNext("");
subscriber.onComplete();
}
catch (Exception ex){
ex.printStackTrace();
}
}
});
}
// --------------------------------------------------------------------------------------
// --------------------------------------------------------------------------------------
[참고 사이트]
// --------------------------------------------------------------------------------------
[raw 폴더 , menu 폴더 , anim 폴더 생성 방법 설명]
https://blog.naver.com/kkh0977/222356039600?trackingCode=blog_bloghome_searchlist
[커스텀 다이얼로그 (custom dialog alert) 팝업창 만들기]
https://blog.naver.com/kkh0977/222590922634?trackingCode=blog_bloghome_searchlist
// --------------------------------------------------------------------------------------
반응형
'Android' 카테고리의 다른 글
Comments